Adding code blocks to the blog
Updating my blog to allow code blocks was fairly simple.
The steps to do this were:
1) Add a code input plugin package and set it up in the CMS (Sanity)
2) Add a package to parse the code input on the front end (Gatsby) and make the serializer component.
Code-input for sanity
The package I decided to use was @sanity/code-input as it is an official tool from the Sanity dev team.
1# Go to sanity directory
2sanity install @sanity/code-input
Once that's completed, go into whatever schema definition for the rich text fields you are using and add
1/* blockContent.js */
2{
3 type: 'code',
4},
Now you'll see the code dropdown here
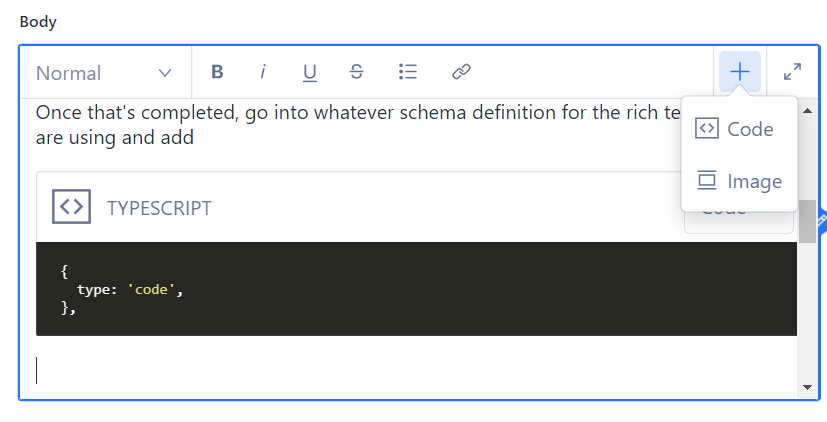
Upon selecting it there is a popup where you can input code into the rich text field.
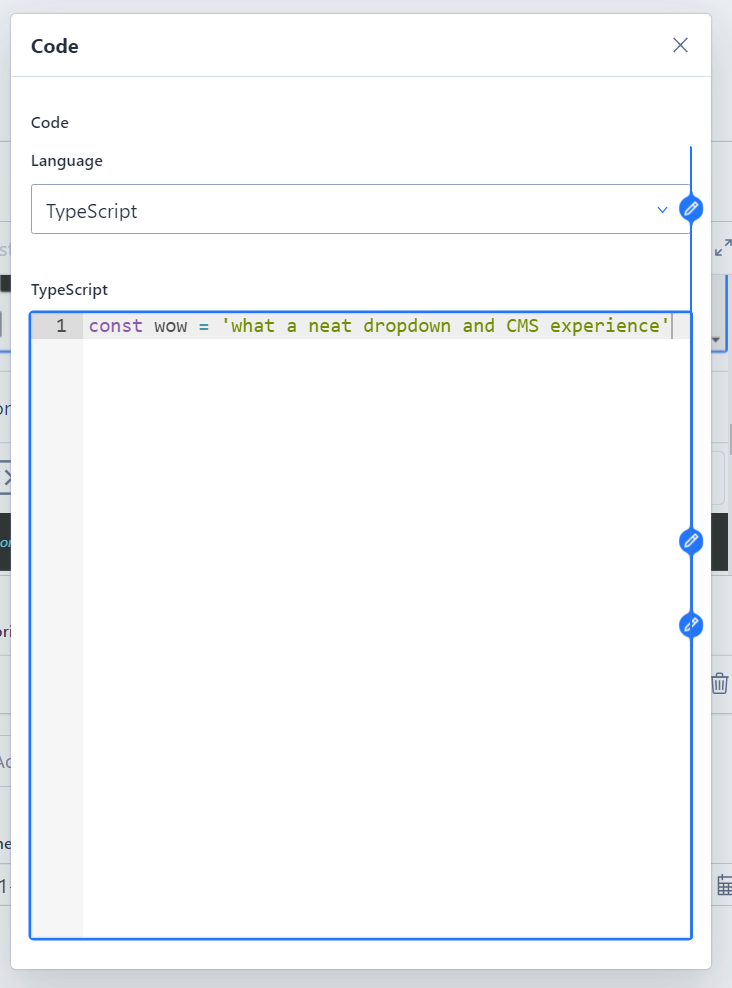
That's it for the CMS side, make sure to deploy the sanity changes.
React Syntax Highlighter
The package for the frontend I decided to use was
because it's the most popular and it has awesome built in styles!
First we need to tell Sanity we want to use a custom Code component:
1/* BlogPost.tsx */
2import Code from './Code'
3/*
4...
5*/
6const serializers = {
7 types: {
8 image: Figure,
9 // New custom serializer for code render
10 code: Code,
11 },
12}
13
14/* Serializer is used on BlockContent as a prop
15...
16<BlockContent
17 serializers={serializers}
18/>
19...
20*/
After that we create the Code component:
1/* Code.tsx */
2import React from 'react'
3import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter'
4import { materialOceanic } from 'react-syntax-highlighter/dist/esm/styles/prism'
5import styled from 'styled-components'
6
7// Override selection styles as orange on orange looks bad
8const SyntaxHighlighterStyles = styled(SyntaxHighlighter)`
9 * {
10 &::selection {
11 background: #accef7;
12 }
13 &::-moz-selection {
14 background: #accef7;
15 }
16 }
17`
18interface Props {
19 node: {
20 code: React.ReactNode
21 language: string
22 }
23}
24
25/**
26 * Code Serializer component for Sanity code-input.
27 */
28const Code: React.FC<Props> = ({ node: { language, code } }) => (
29 <>
30 <SyntaxHighlighterStyles
31 language={language || 'text'}
32 showLineNumbers
33 style={materialOceanic}
34 >
35 {code}
36 </SyntaxHighlighterStyles>
37 </>
38)
39
40export default Code
41
And that's it! Now the blog has a new Code element in the CMS, and Syntax Highlighting on the front end.
Here's the commit on my github: https://github.com/jhuan015/personal-website/commit/ae892f84c67cf11e87f0ab95f2e352c403c4184b